Themes allow you to customise the appearance of your charts. They provide defaults for different properties of the chart that will be used unless overridden by the chart options.
Using Stock Themes Copy Link
Every chart uses the 'ag-default'
theme unless configured otherwise.
AgCharts.create({
theme: 'ag-default', // optional, implied
//...
});
Financial Charts use the ag-financial
and ag-financial-dark
themes only.
The following themes are provided out-of-the-box:
type AgChartThemeName =
| 'ag-default'
| 'ag-default-dark'
| 'ag-sheets'
| 'ag-sheets-dark'
| 'ag-polychroma'
| 'ag-polychroma-dark'
| 'ag-vivid'
| 'ag-vivid-dark'
| 'ag-material'
| 'ag-material-dark';
In the example below, you can click the buttons to change the theme used in the chart.
Making Custom Themes Copy Link
You can create your own theme, which builds upon an existing theme and allows you to change as many or as few properties as you like.
A custom theme is an object with the following optional properties:
baseTheme
- The name of the theme to base this theme upon (if not specified, the'ag-default'
theme is used).palette
- The palette to use, replaces the palette of the base theme.params
- The theme parameters to set global styles.overrides
- The object to be merged with the base theme's defaults and override them.
See the Themes API page for the complete options structure.
Palette Copy Link
A palette is the array of colours used for the data, for example the bars in a bar series chart.
If there are more groups of data than colours in the palette, the colours will be repeated.
const myTheme = {
palette: {
fills: ['#006f9b', '#ff7faa', '#00994d', '#ff8833', '#00a0dd'],
strokes: ['#003f58', '#934962', '#004a25', '#914d1d', '#006288'],
},
};
Parameters Copy Link
The params
object is used to set styles across the whole chart in one place.
- Set the font with
fontFamily
andfontSize
. - Use
foregroundColor
,backgroundColor
andaccentColor
to set the main colours on the chart. All other colours will default to a mix of these three. - Use specific params to adjust tooltips, menus, panels, toolbars, buttons and text inputs, for example
tooltipBackgroundColor
. Please see the Themes API reference for the full list of available options.
const myTheme = {
palette: {
fills: ['#006f9b', '#ff7faa', '#00994d', '#ff8833', '#00a0dd'],
strokes: ['#003f58', '#934962', '#004a25', '#914d1d', '#006288'],
},
params: {
foregroundColor: '#262a33',
backgroundColor: '#fff1e5',
accentColor: '#0d7680',
fontFamily: [{ googleFont: 'DM Serif Text' }, 'Georgia', 'sans-serif'],
fontSize: 14,
tooltipBackgroundColor: '#fff7ef',
tooltipTextColor: '#262a33',
},
};
In the above example:
- The three main colours are defined with some additional specific colours for chrome elements.
- All text elements are set to the same font family.
- All text, except the titles and footer, are set to the same font size.
Overrides Copy Link
The overrides
object is similar in its structure to the chart's options and is used as follows:
- Items in the
overrides.common
object are applied to all chart types. - Items in the series specific object (e.g.
overrides.line
), are applied to charts of that series type. - Series specific properties are found in the
series
object of the series type (e.g.overrides.line.series
). - The
axes
config contains one object for each axis type.
const myTheme = {
palette: {
fills: ['#5C2983', '#0076C5', '#21B372', '#FDDE02', '#F76700', '#D30018'],
strokes: ['black'],
},
overrides: {
common: {
title: {
fontSize: 24,
},
},
bar: {
series: {
label: {
enabled: true,
color: 'black',
},
strokeWidth: 1,
},
},
},
};
In the above example:
- A different palette of colours and strokes is provided for all series to use.
- The font size of the title is increased.
- The
bar
series has specific label and stroke options set.
Advanced Custom Theme Copy Link
const myTheme: AgChartTheme = {
palette: {
fills: ['#006f9b', '#ff7faa', '#00994d', '#ff8833', '#00a0dd'],
strokes: ['#003f58', '#934962', '#004a25', '#914d1d', '#006288'],
},
params: {
foregroundColor: '#262a33',
backgroundColor: '#fff1e5',
accentColor: '#0d7680',
chromeBackgroundColor: '#fff7ef',
chromeTextColor: '#262a33',
fontFamily: 'Georgia, serif',
fontSize: 14,
},
overrides: {
common: {
title: {
fontSize: 24,
},
padding: {
left: 70,
right: 70,
},
axes: {
category: {
line: {
width: 4,
},
},
number: {
line: {
width: 2,
},
},
},
},
line: {
series: {
marker: {
shape: 'circle',
},
},
},
bar: {
series: {
label: {
enabled: true,
color: 'white',
},
},
},
pie: {
padding: {
top: 40,
bottom: 40,
},
legend: {
position: 'left',
},
series: {
calloutLabel: {
enabled: true,
},
calloutLine: {
colors: ['#881008'],
},
},
},
},
};
In the above example:
- A different palette of colours and strokes is provided for all series to use.
- Some
param
global styles are specified. - Some
common
overrides are specified which apply to all chart types. - Different axes overrides are specified for
category
andnumber
axes types. - Different series specific overrides are specified for
bar
,line
andpie
series.
Figma Design System Copy Link

The AG Grid Figma Design System contains AG Charts components and templates to aid you in mocking up and theming AG Charts applications.
The design system contains UI components such as tooltips, menus, navigators, and financial charts toolbars. There are also Figma assets to aid in the design of AG Charts colour palettes. In addition, we supply a full range of components for mocking up AG Grid integrated charts applications. Due to the limitations of Figma itself, we do not supply assets to mock up chart series.
To get started with the Figma Design System, visit the AG Grid Figma Design System Figma community page and click the "Open in Figma" button.
We supply the AG Grid Design System as a Figma community file. You will use the file by duplicating it to your Figma workspace. While we regularly update the AG Grid Design System, your duplicated files will not receive any updates automatically.
For more information about how Figma community files function, please review the Figma help pages on community files.
Figma Documentation & Video Tutorials Copy Link
You’ll find comprehensive documentation for the design system right within the Figma file. We have guides for getting started and pre-made chart templates. Further detailed information about how each chart component works is also within the Figma file.
Watch our video on Theming AG Charts in Figma with Variables and Tokens. We also have a complete playlist of in-depth videos diving deeper into working with the AG Grid Figma design system.
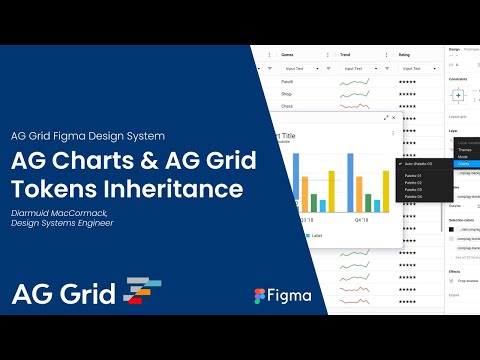
Figma Support Copy Link
AG Charts Enterprise customers can request support or suggest features and improvements via Zendesk. Community users can file bug reports via our design system GitHub repo issues.